UPS CityValidation is function that differs quite a bit from the UPS Address Validation function. UPS CityValidation takes two arguments, the City and the State, and provides you with the highest and lowest ZIP Code in that area. It can also identify misspellings or State/City mix-ups and suggest corrections. This function is a simple ColdFusion function that calls the UPS city validation API and fetches the results.
Just like the UPS Address Verification function, you need an UPS API access license to use the UPS CityValidation function. You can register for that in here. I have set those details in my application file as application.UPSLicense, application.UPSUserId and application.UPSPassword.
Here is the function:
1: <cffunction name="cityValidation" access="remote" returntype="struct" output="true"> 2: <cfargument name="city" type="string" required="yes"> 3: <cfargument name="state" type="string" required="yes"> 4: <cfargument name="License" type="string" required="No" default="#application.UPSLicense#"> 5: <cfargument name="UserId" type="string" required="No" default="#application.UPSUserId#"> 6: <cfargument name="Password" type="string" required="No" default="#application.UPSPassword#"> 7: 8: <cfset var local = StructNew()> 9: 10: <cfsavecontent variable="local.reqxml"><cfoutput> 11: <?xml version=
"1.0"?>
12: <AccessRequest xml:lang="en-US"> 13: <AccessLicenseNumber>#arguments.License#
</AccessLicenseNumber> 14: <UserId>#arguments.UserId#
</UserId> 15: <Password>#arguments.Password#
</Password> 16: </AccessRequest> 17: <?xml version=
"1.0"?>
18: <AddressValidationRequest xml:lang="en-US"> 19: <Request> 20: <TransactionReference> 21: <CustomerContext>Customer Data</CustomerContext>
22: <XpciVersion>1.0001
</XpciVersion> 23: </TransactionReference> 24: <RequestAction>AV
</RequestAction> 25: </Request> 26: <Address> 27: <City>#arguments.city#
</City> 28: <StateProvinceCode>#arguments.state#
</StateProvinceCode> 29: </Address> 30: </AddressValidationRequest> 31: </cfoutput></cfsavecontent> 32: <cfhttp url="https://wwwcie.ups.com/ups.app/xml/AV" method="post" result="result"> 33: <cfhttpparam type="xml" name="data" value="#local.reqxml#"> 34: </cfhttp> 35: 36: <cfset local.results = XMLParse(result.Filecontent)> 37: <cfset local.out = StructNew()> 38: <cfset local.out.validation = "True"> 39: <cfset local.out.city = ""> 40: <cfset local.out.state = ""> 41: <cfset local.out.PostalCodeLowEnd = ""> 42: <cfset local.out.PostalCodeHighEnd = ""> 43: 44: <cfif not IsDefined('local.results.AddressValidationResponse.AddressValidationResult')> 45: <cfset local.out.validation = "False"> 46: <cfelse> 47: <cfif (not local.results.AddressValidationResponse.AddressValidationResult.Address.City.XmlText eq trim(arguments.city)) or 48: (not local.results.AddressValidationResponse.AddressValidationResult.Address.StateProvinceCode.XmlText eq trim(arguments.state))> 49: <cfset local.out.validation = "Corrected"> 50: <cfset local.out.city = local.results.AddressValidationResponse.AddressValidationResult.Address.City.XmlText> 51: <cfset local.out.state = local.results.AddressValidationResponse.AddressValidationResult.Address.StateProvinceCode.XmlText> 52: </cfif> 53: <cfset local.out.PostalCodeLowEnd = local.results.AddressValidationResponse.AddressValidationResult.PostalCodeLowEnd.XmlText> 54: <cfset local.out.PostalCodeHighEnd = local.results.AddressValidationResponse.AddressValidationResult.PostalCodeHighEnd.XmlText> 55: </cfif> 56: <cfreturn local.out> 57: </cffunction> If the City and State you have provided is correct, it will return VALIDATION = TRUE and ZIP Code Values.
For example,
cityValidation('Bronx', 'NY')returns this:
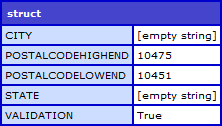
If UPS fixed your city or state, the value for the VALIDATION will be "CORRECTED,"
as I’ve show with the wrong State And City combination I provided here:
cityValidation('Bronx', 'NJ')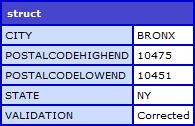
If the validation failed altogether, as with the fake city I provided here:
cityValidation('FAKE', 'NJ')It will return VALIDATION = False
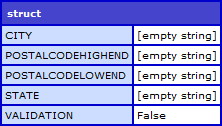
Posted by Saman W Jayasekara at Friday 15 October 2010 01:47 PM
.
Shipping API
.
ColdFusion